Home > backend > Cross-Origin Resource Sharing (CORS): What You Need To Know.
Cross-Origin Resource Sharing (CORS): What You Need To Know.
CORS has become an essential component of modern web development, yet it remains a source of confusion and frustration for many developers.
By :Thomas Inyang🕒 20 May 2025
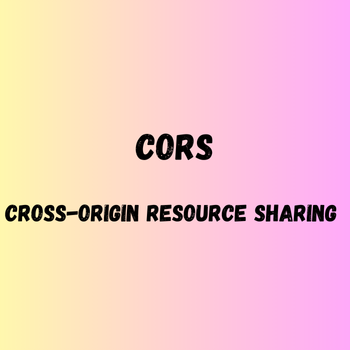
Introduction
In an interconnected web environment, applications frequently need to request resources from different domains, servers, and ports. However, web browsers implement a security feature called the Same-Origin Policy that restricts how documents or scripts from one origin can interact with resources from another origin. This is where Cross-Origin Resource Sharing (CORS) enters the picture.
CORS has become an essential component of modern web development, yet it remains a source of confusion and frustration for many developers. Whether you're encountering cryptic CORS errors in your browser console or trying to understand why your API calls aren't working as expected, this comprehensive guide will demystify CORS, explain its purpose, and provide practical solutions to common CORS-related challenges.
At the end of this article, you'll have a thorough understanding of the following:
- What CORS is, why it exists, and how it works.
- How to implement CORS correctly using different backend programming languages.
What is CORS? Understanding the Fundamentals
This is a security mechanism built into browsers that allows servers to specify which origins (domains, schemes, or ports) are permitted to access resources on the server by adding HTTP headers.
When you visit a website, your browser makes various requests for resources like images, stylesheets, scripts, and data. If these resources are hosted on the same domain (origin) as the website you're visiting, there's no issue. However, when a web application attempts to request resources from a different origin, the Same-Origin Policy kicks in.
The Same-Origin Policy is a critical security measure implemented by web browsers that restricts how a document or script loaded from one origin can interact with resources from another origin. Without this policy, malicious websites could freely access data from other websites you're logged into, potentially leading to data theft and other security breaches.
CORS provides a controlled relaxation of this restriction, allowing servers to explicitly indicate which origins are permitted to access their resources. It's important to understand that CORS is not a security feature that prevents unauthorized access to resources; rather, it's a mechanism that enables browsers to safely allow cross-origin requests that would otherwise be forbidden by the Same-Origin Policy.
What Does CORS Do?
It enables servers to specify which domains are allowed to access their resources, handle both simple requests and those that require preflight checks, preserve the security intentions of the Same-Origin Policy, and make it possible to build web applications that integrate resources from multiple domains.
Understanding what CORS does is essential for both frontend and backend developers working on web applications that need to share resources across different domains.
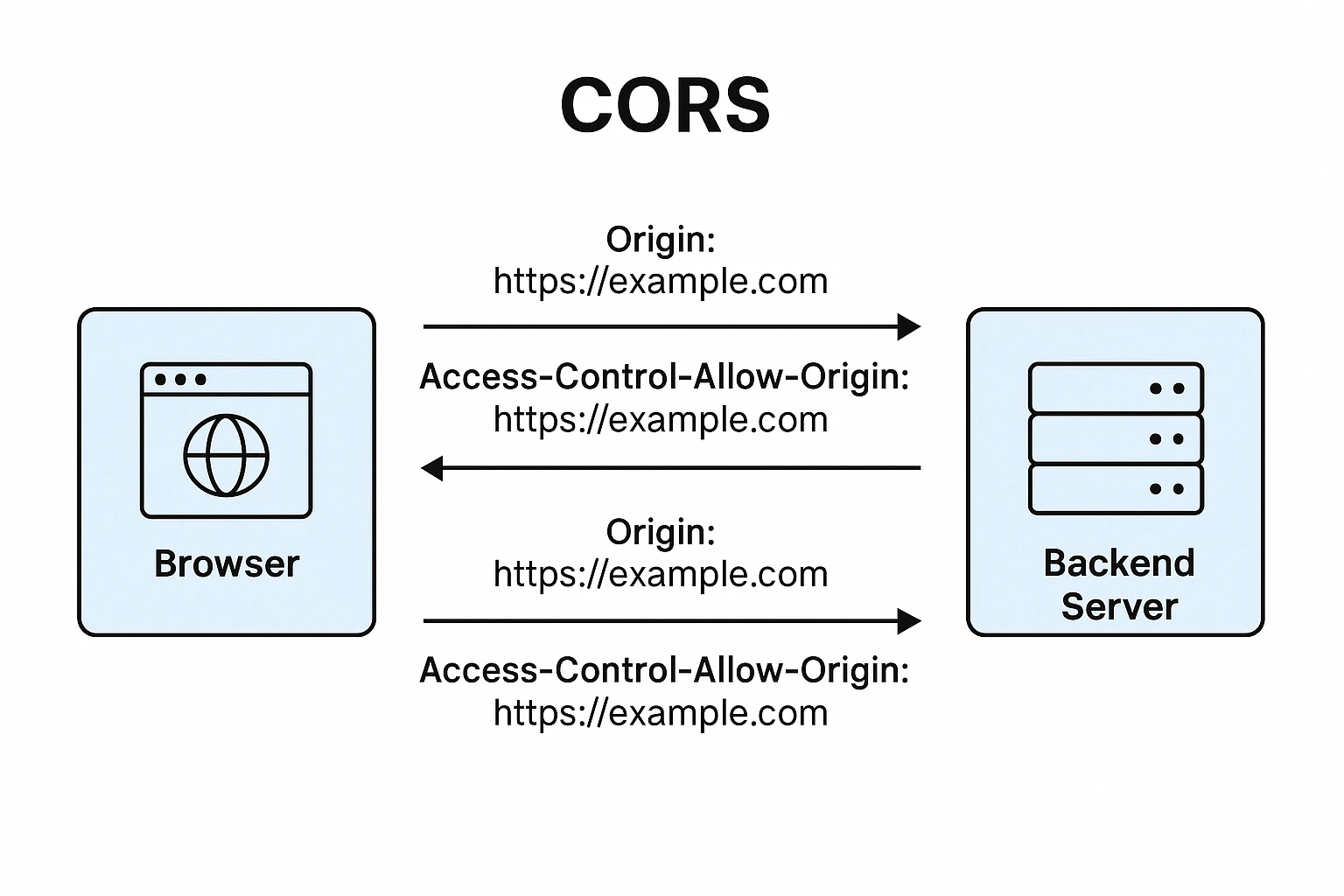
The History and Development of CORS
CORS was developed by the World Wide Web Consortium (W3C) as part of the Web Application Working Group. The initial specification was proposed around 2005 as web applications became increasingly sophisticated and the need to share resources across domains grew more common.
See Also: Get Started With Web Development
The CORS specification evolved over several years, with contributions from browser vendors, security experts, and web developers. It was officially standardized as a W3C Recommendation in January 2014, though browsers had already begun implementing aspects of it before the final standardization.
Who Controls CORS?
No single entity "controls" CORS. The specification is maintained by the W3C, but its implementation is handled by individual browser vendors. It means that while the specification provides guidelines, the actual behavior might vary slightly across different browsers.
On the server side, CORS is controlled by the server administrators, who determine which origins are allowed to access their resources by configuring the appropriate HTTP headers. This decentralized approach means that each server owner decides their own CORS policy based on their security requirements and the needs of their applications.
What Problem Does CORS Solve?
Before CORS, developers faced significant limitations when attempting to build web applications that needed to interact with resources from multiple origins. The Same-Origin Policy, while important for security, became a roadblock for legitimate use cases such as:
- APIs serving multiple clients: Modern web services often need to provide data to applications hosted on different domains.
- Microservices architecture: Applications split across multiple domains or subdomains need to communicate with each other.
- Content delivery networks (CDNs): Resources loaded from CDNs are served from different domains than the main application.
- Third-party integrations: Applications incorporating functionality from external services need to exchange data with those services.
CORS solved these problems by providing a standardized way for servers to declare which origins are permitted to access their resources, all while maintaining the security benefits of the Same-Origin Policy.
How CORS Works: The Technical Details
Understanding the technical mechanisms behind CORS is crucial for effectively implementing and troubleshooting it in your applications. Here is a breakdown of how CORS actually works behind the scenes.
CORS is not a standalone protocol but rather an extension of HTTP. It works by adding new HTTP headers that allow servers to describe the set of origins that are permitted to read information from a web server. These headers form part of the HTTP protocol and are interpreted by browsers to enforce access controls.
The CORS Request Flow
When a browser makes a cross-origin request, the CORS mechanism typically follows one of two flows:
- Simple Requests: These don't trigger a preflight check and are sent directly to the server with an Origin header that indicates where the request is coming from.
- Preflighted Requests: More complex requests first send an OPTIONS HTTP request (the "preflight") to determine whether the actual request is safe to send.
Simple vs. Preflighted Requests
A request is considered simple when it meets all of the following criteria:
- Uses only the methods GET, HEAD, or POST.
- If using POST, only uses content types of application/x-www-form-urlencoded, multipart/form-data, or text/plain
- Does not set custom headers.
All other requests are considered "non-simple" and require a preflight check. This consideration includes requests using methods like PUT or DELETE, those with custom headers, or those using content types like application/json.
Key CORS Headers
The most important headers in CORS communication include:
- Request headers: the Origin Indicates where the cross-origin request is coming from
- Response headers such as:
- Access-Control-Allow-Origin: Specifies which origins can access the resource.
- Access-Control-Allow-Methods: Specifies the HTTP methods allowed when accessing the resource.
- Access-Control-Allow-Headers: Specifies which headers can be used in the actual request.
- Access-Control-Max-Age: Indicates how long the results of a preflight request can be cached.
- Access-Control-Allow-Credentials: Indicates whether the browser should include credentials (like cookies) with the request.
Understanding these headers is essential for properly configuring CORS on your server.
The Benefits and Risks of Implementing CORS
Understanding both the advantages and potential drawbacks of CORS is essential for making informed decisions about how to implement it in your applications.
CORS offers several significant benefits for modern web development:
- CORS allows web applications to safely access resources hosted on different domains, which is essential for distributed architectures and third-party integrations.
- By providing a controlled relaxation of the Same-Origin Policy, CORS preserves security while enabling necessary cross-domain interactions.
- CORS provides a well-defined, browser-supported standard for handling cross-origin requests, eliminating the need for less secure workarounds.
- CORS facilitates microservices, API-driven development, and other modern approaches that involve components distributed across multiple domains.
- By enabling direct communication between frontend applications and various backends, CORS helps create more integrated and responsive user experiences.
What Is the Risk of CORS?
While CORS itself is a security mechanism, improper implementation can introduce the following risks:
- Overly permissive configurations: Setting Access-Control-Allow-Origin: * allows any website to access your resources, which might not be appropriate for sensitive data or operations.
- Credential exposure: Combining Access-Control-Allow-Origin: * with Access-Control-Allow-Credentials: true can potentially expose sensitive user information to unauthorized domains.
- Header leakage: Exposing too many headers through Access-Control-Expose-Headers might reveal information that should remain private.
- Origin spoofing in internal networks: In some network configurations, attackers might be able to spoof the Origin header, though this is typically only an issue in internal networks.
- Complexity leading to misconfiguration: The complexity of CORS can lead to misconfigurations that either block legitimate requests or allow unauthorized access.
What Does CORS Protect Against?
While CORS is often misunderstood as a complete security solution, it primarily protects against the following:
- Cross-site data theft: CORS helps prevent malicious websites from reading sensitive data from other sites you're logged into.
- Unauthorized state-changing requests: By requiring preflight checks for non-simple requests, CORS adds an additional layer of protection against cross-site request forgery for certain types of requests.
It's important to note that CORS does not replace other security measures like authentication, authorization, CSRF tokens, and content security policies. It works alongside these measures to create a comprehensive security strategy.
Implementing CORS: Backend vs. Frontend Considerations
Properly implementing CORS requires understanding both backend and frontend aspects, as they work together to enable secure cross-origin requests.
Is CORS Backend or Frontend?
CORS is primarily implemented on the backend server, which must send the appropriate HTTP headers to allow or restrict access. However, the enforcement of CORS policies happens on the frontend (in the browser), which checks these headers and either allows or blocks requests accordingly.
This dual nature means that backend developers must correctly configure their servers to send appropriate CORS headers based on the application's security requirements. While frontend developers need to understand CORS to properly structure their requests and troubleshoot issues when cross-origin requests fail.
Setting Up CORS on Different Backend Technologies
Here's how to implement basic CORS support in several popular backend frameworks:
Node.js with Express:
Python with Flask:
Java with Spring Boot:
PHP:
Installing CORS capability typically involves adding middleware or configuration to your server:
- For Node.js/Express: Install the cors package with npm install cors
- For Python/Flask: Install flask-cors with pip install flask-cors
- For Java/Spring: Spring Boot includes CORS support by default
- For PHP: No installation needed, just add the appropriate headers to your responses
- For .NET Core: CORS middleware is included in the framework.
For cloud storage services like Azure Storage, AWS S3, or Google Cloud Storage, CORS settings are typically configured through the service's management console or API:
Azure Storage Account:
- Navigate to your storage account in the Azure portal
- Select "Resource sharing (CORS)" under "Settings"
- Add a new CORS rule specifying allowed origins, methods, headers, etc.
- Save your changes
AWS S3:
- Open the S3 console and select your bucket
- Go to "Permissions" tab
- Scroll to the "Cross-origin resource sharing (CORS)" section
- Click "Edit" and add your CORS configuration in JSON format
- Save the changes
Google Cloud Storage:
- Use the gsutil command-line tool or REST API
- Create a JSON file with your CORS configuration
- Apply it using gsutil cors set corsconfig.json gs://your-bucket
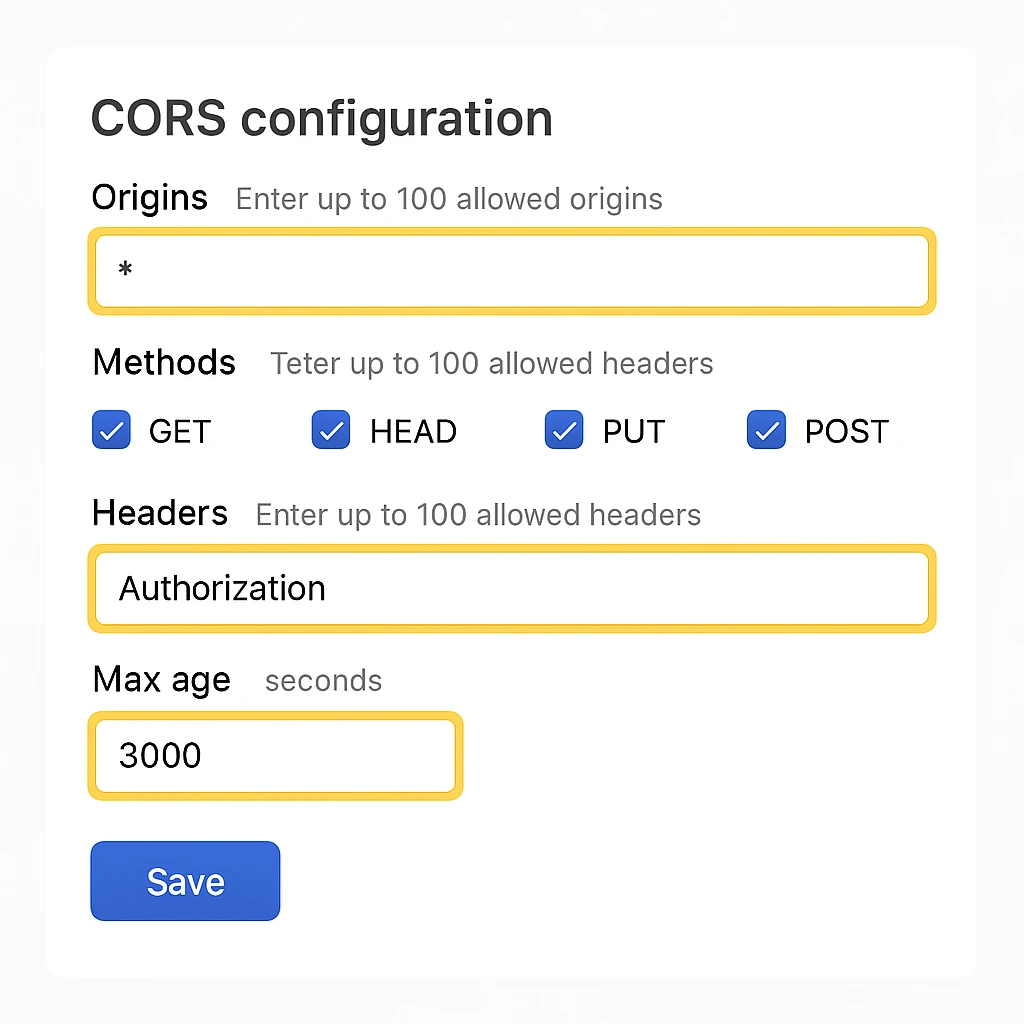
Common CORS Errors and How to Fix Them
Despite its importance, CORS is often a source of frustration for developers due to the various errors that can occur. Understanding these errors and their solutions is key to smooth development.
CORS errors typically occur when:
- The server doesn't include necessary CORS headers in its responses
- The origin making the request is not included in the allowed origins
- The request method or headers aren't allowed by the server's CORS policy
- Credentials are included but not properly configured on both ends
- The preflight response is incorrect or missing required headers
- Network issues prevent the preflight request from completing successfully
- Intermediaries like proxies or CDNs strip or modify CORS headers
Here's a systematic approach to fixing CORS errors:
- Identify the specific error: Look at the browser console for detailed error messages that indicate which aspect of CORS is failing.
- Check server configuration: Ensure your server is correctly configured to send the appropriate CORS headers for your use case.
- Verify allowed origins: Make sure the origin of your frontend application is included in the Access-Control-Allow-Origin header.
- Check request complexity: Remember that complex requests trigger preflight checks. Ensure your server correctly responds to OPTIONS requests.
- If your requests include credentials (cookies, HTTP authentication), ensure Access-Control-Allow-Credentials: true is set and that Access-Control-Allow-Origin specifies an exact origin (not the wildcard *).
- Use browser developer tools to examine both the preflight request (if applicable) and the actual request to see what headers are being sent and received.
- Consider environmental factors: Different environments (development, staging, production) might have different origins or configurations.
Common CORS Error Solutions
For "No 'Access-Control-Allow-Origin' header is present" errors:
// On your server, add:
// Or for development:
For preflight request issues:
// Ensure your server responds properly to OPTIONS requests
For credential-related issues:
// Server-side
While properly fixing CORS issues by configuring your server is the recommended approach, there are temporary workarounds for development:
- Configure your development server to proxy requests to the API, eliminating the cross-origin nature of the requests.
- Browser extensions: Extensions like "CORS Unblock" or "Allow CORS" can disable CORS checks in the browser during development.
- CORS Anywhere service: Services like CORS Anywhere can proxy your requests and add the necessary CORS headers.
- Disable web security in the browser: Launch Chrome with the --disable-web-security flag (NEVER do this for regular browsing, only for testing in a dedicated browser instance).
Remember that these bypass methods are not suitable for production and should only be used during development.
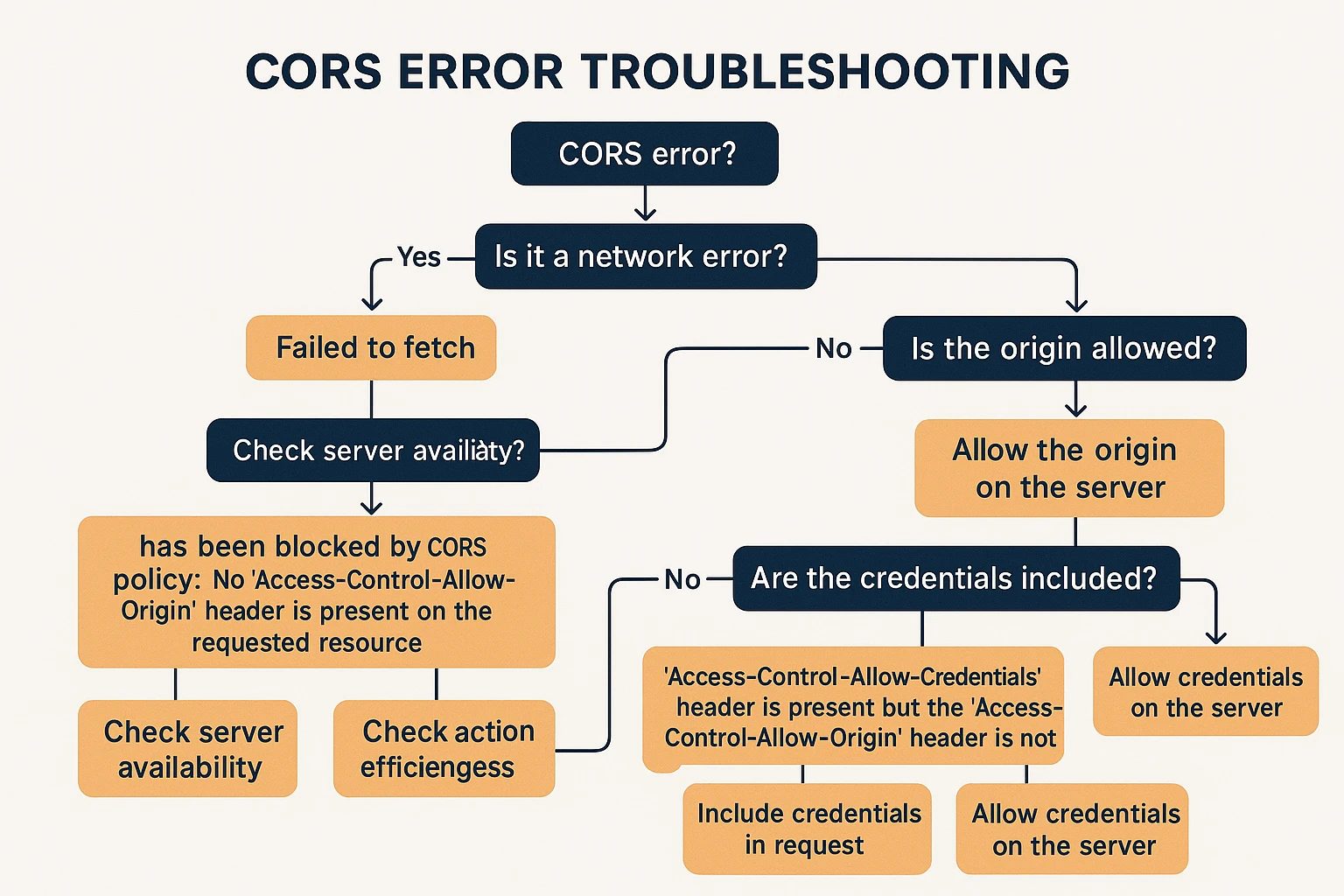
Advanced CORS Concepts and Best Practices
As you become more familiar with CORS, understanding these advanced concepts and best practices will help you implement more secure and efficient cross-origin resource sharing.
What is the Difference Between CSRF and CORS?
CORS (Cross-Origin Resource Sharing) and CSRF (Cross-Site Request Forgery) are often confused but address different security concerns:
- CORS is a security feature that relaxes the Same-Origin Policy to allow legitimate cross-origin requests. It's about giving servers control over who can access their resources.
- CSRF is an attack where a malicious site tricks a user's browser into making unwanted requests to a site where the user is authenticated. CSRF protection prevents these unwanted actions.
Interestingly, CORS can actually help prevent some CSRF attacks by requiring preflight checks for complex requests. However, simple GET requests can still be vulnerable, which is why dedicated CSRF protection (like tokens) is still necessary.
Best Practices for CORS Implementation
- Be specific with origins: Instead of using Access-Control-Allow-Origin: *, specify exactly which origins are allowed to access your resources.
- Only expose headers that are necessary for the client application using Access-Control-Expose-Headers.
- Cache preflight responses: Use Access-Control-Max-Age to reduce the number of OPTIONS requests and improve performance.
- Be careful with credentials: When allowing credentials, always specify exact origins and never use the wildcard *.
- Return appropriate HTTP status codes and error messages when CORS checks fail.
- Regularly audit your CORS configuration: Ensure it reflects your current security requirements and doesn't include outdated or unnecessary permissions.
- Use CORS in conjunction with other security measures: CORS is just one layer of security and should be combined with proper authentication, authorization, and input validation.
Testing and Debugging CORS Configurations
Properly testing and debugging CORS configurations is essential to ensure that your cross-origin requests work as expected while maintaining security.
How to Run a Browser Without CORS?
For development and testing purposes, you can run browsers with CORS disabled:
Chrome: chrome --disable-web-security --user-data-dir="[some directory here]"
Firefox: Install the "CORS Everywhere" extension
Edge: edge --disable-web-security --user-data-dir="[some directory here]"
Safari: In the Develop menu, enable "Disable Cross-Origin Restrictions."
Important Security Warning: Running browsers with security features disabled makes them vulnerable to various attacks. Only use these options for development and testing in a controlled environment, never for regular browsing.
How to Use CORS Anywhere and Proxies
CORS Anywhere is a NodeJS proxy that adds CORS headers to the proxied request.
How it works:
- Instead of requesting a resource directly, you request it through the CORS Anywhere proxy.
- The proxy forwards your request to the target server.
- When the proxy receives the response, it adds the necessary CORS headers before sending it back to your application.
Here's how to use it:
- Use the public instance (not recommended for production):
- Host your own instance for development:
Other proxy options include:
- Setting up a development proxy in frameworks like React, Vue, or Angular
- Using server-side proxies in your backend code
- Configuring a proxy in your web server (Nginx, Apache, etc.)
Important considerations:
- CORS Anywhere is primarily intended for development and testing, not production use.
- The public CORS Anywhere instance has usage limitations.
- For production applications, it's better to properly configure CORS on your own server or use a server-side proxy.
FAQ on CORS in Modern Web Development Scenarios
CORS impacts various aspects of modern web development. Understanding these specific scenarios will help you apply CORS correctly in different situations.
Does CORS Apply to API?
Yes, CORS absolutely applies to APIs that are accessed via browsers. In fact, APIs are one of the most common scenarios where CORS is relevant:
- Browser-based applications making requests to APIs on different domains must comply with CORS.
- Server-to-server API requests are not subject to CORS because they don't involve a browser.
- Mobile apps making API requests generally don't deal with CORS directly (unless they're using a web view), but the same principles of origin security might be applied through other mechanisms.
For web APIs that need to be accessible from browser-based clients on different domains, proper CORS configuration is essential.
Does CORS Work with IP Addresses?
Yes, CORS works with IP addresses as origins, but there are some considerations:
- You can specify IP addresses in your CORS configuration: Access-Control-Allow-Origin: http://192.168.1.5:3000.
- Remember that http://127.0.0.1 and http://localhost are considered different origins by browsers, even though they resolve to the same location.
- When using IP addresses for local development, make sure to include the protocol and port in your CORS configuration.
- For production environments, using domain names is generally preferred over IP addresses.
Does CORS Work on HTTP?
Yes, CORS works on both HTTP and HTTPS connections, but with some important security considerations:
- Modern browsers may impose additional restrictions when mixing HTTP and HTTPS in cross-origin requests.
- If your site is served over HTTPS, browsers might block mixed content, including HTTP requests even if they would otherwise be allowed by CORS.
- Some CORS features, particularly those involving credentials, are more secure when both origins use HTTPS.
For the best security and to avoid potential issues, it's recommended to use HTTPS for both the requesting site and the resource server.
Conclusion
Understanding Cross-Origin Resource Sharing (CORS) is essential for modern web developers working with distributed systems and APIs. Throughout this guide, we've explored what CORS is, how it works, and how to implement it correctly in various scenarios.
Here are key takeaways from this article:
- CORS is a security feature, not a bug. It enables controlled access to resources across different origins while maintaining the security benefits of the Same-Origin Policy.
- Implementation happens on the server side, where you configure which origins, methods, and headers are allowed. The browser enforces these policies on the client side.
- Different request types are handled differently. Simple requests go directly to the server with an Origin header, while more complex requests require a preflight OPTIONS request.
- Proper CORS configuration balances security and functionality. Be specific about which origins and methods you allow, and be careful when handling credentials.
- CORS errors are common but solvable. By understanding the specific error and systematically checking your configuration, you can resolve most CORS issues quickly.
- Testing and debugging tools are available. From browser developer tools to specialized proxy services, you have many options for troubleshooting CORS issues.
By mastering CORS, you'll be better equipped to build secure, flexible web applications that can safely communicate across different domains. Remember that CORS is just one piece.
Please Share.