Home > software_development > How Gin Framework handles Cookies in Golang.
How Gin Framework handles Cookies in Golang.
Use Gin-Golang web framework to set and get HTTP Cookies.
By :Thomas Inyang🕒 25 May 2025
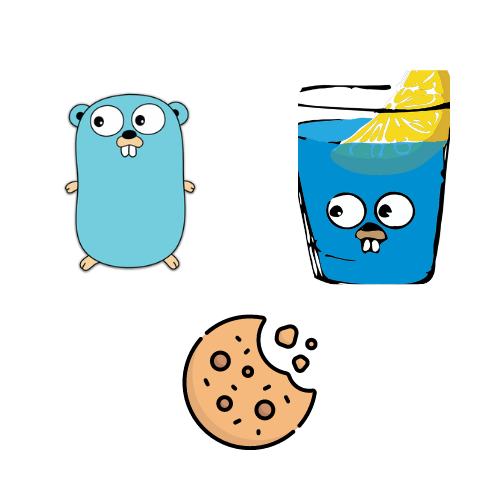
As a backend developer, thoughts of how websites remember your last login session, store your last online shopping search, or retain items in your shopping cart must have crossed your mind. Well, that is the work of a Cookie (HTTP Cookie) and this Cookie differs from the regular cookie we eat.
In this post, you will learn the following about Hypertext Transfer Protocol (HTTP) cookies in Gin - Golang web framework:
- What HTTP cookies are and why they're essential for modern web applications.
- The different types of cookies and their specific use cases
- How to implement cookies in Gin, from basic setup to production-ready code
- Security best practices to protect your users and their data.
Prerequisite
- Install Golang
- Familiar with HTTP request methods.
What are Cookies (HTTP cookies)?
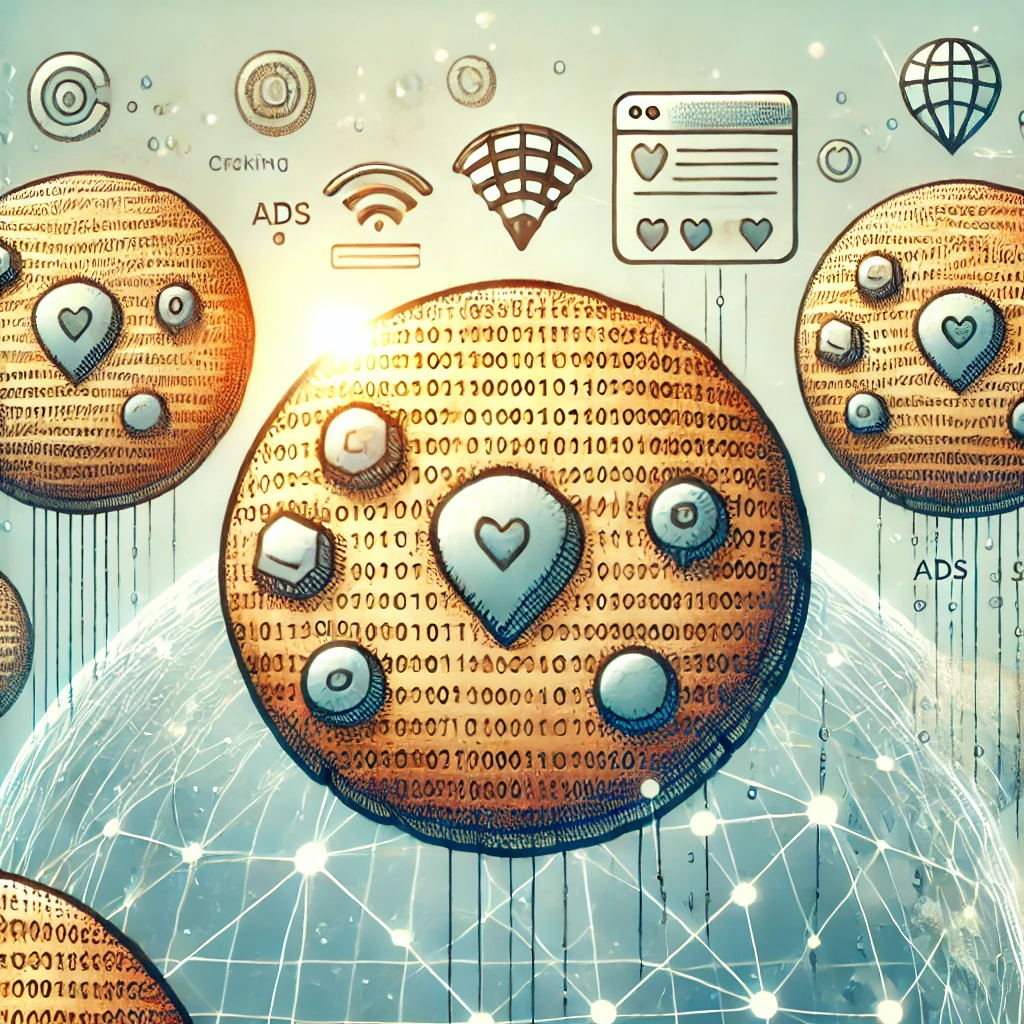
Cookies are the bridge that allows websites to maintain state across multiple requests. These small pieces of data are created by the server and stored on the user's computer, allowing for persistent information between browsing sessions.
What Exactly Are HTTP Cookies?
HTTP cookies (sometimes called browser or internet cookies) are tiny data packets stored on a user's device when they visit a website. They serve as a memory mechanism, allowing servers to recognize returning visitors and maintain stateful information in an inherently stateless protocol.
Unlike localStorage or sessionStorage, cookies are automatically sent with every HTTP request to the domain that created them, making them ideal for authentication and session management.
Why Are Cookies Needed?
Cookies are used to solve several critical problems in web development:
- Session Management: They enable authentication and user session tracking
- User Preferences: They store user settings and choices
- Tracking: They collect data about user behavior and interactions.
What Are The Types of Cookies
There are different types of cookies that help solve specific needs, such as:
- Session Cookies: These are temporary cookies that exist only until the browser is closed
- Persistent Cookies: They are long-lived cookies that remain on the user's device for a specified period.
- Secure Cookies: Cookies that are only transmitted over encrypted HTTPS connections.
- HttpOnly Cookies: With this, your stored cookies cannot be accessed by JavaScript, protecting against XSS attacks
- SameSite Cookies: It controls whether they are sent with cross-site requests
Introduction to Golang and Gin for Cookie Management
A brief understanding of Golang with Gin as an excellent choice for modern web applications.
Why Go (Golang)?
It is a statically typed programming language developed and maintained by Google, and popular among backend developers.
It has a good performance comparable to C++ with the simplicity of Python, built-in concurrency support through go routine, a strong standard library and thriving ecosystem, and excellent support for cloud infrastructure and microservices
Many industry leaders including Google, Uber, Dropbox, and Twitch use Go for their backend services, making it a highly sought-after skill in today's job market.
Why Gin?
It is a web framework written in Go that offers lightning-fast performance that is up to 40 times faster than comparable frameworks like Martini with a Minimalist design ( features you need). It has excellent middleware support for authentication and logging. Also, it is easy to handle request routing.
See Also: What You Need To Know About NodeJs for Backend Development.
For cookie management specifically, Gin provides simple yet powerful APIs that make implementing cookies straightforward while maintaining security.
See also: Implement local module in Golang
Set Cookies with Gin in Golang.
Install the Gin package in the directory you want to set up the cookies functions.
For this example, you will set a Session Cookie - authentication, using the user's email as the value.
Development only sample.
In this code,
- "auth-cookie" is the name of the cookie.
- emailToken is the value of the cookie.
- 30 is the MaxAge (expiration) of the cookie in seconds (in this case 30 seconds).
- "/" is the path for which the cookie is valid (the root path).
- "localhost" is the domain for which the cookie is valid.
- false is the boolean condition means that the cookie can be transmitted over HTTP. Use this during development only.
- true is the boolean condition means that the cookie should be accessible only through HTTP and not Javascript. This is known as the HttpOnly flag.
Production only sample.
In this code, the following changes were made:
- jwtToken: This is the value of the returned jwt token.
- 300: MaxAge, in this case, 5 minutes.
- "hostedDomainUrl": the hosted project domain url.
- true: the boolean condition is changed from false to true, which means it cannot be transmitted over HTTP.
- gincookie.SameSiteStrictMode: this rule prevents CSRF attacks.
Let's see how to retrieve the auth cookie.
Get Cookies with Gin in Golang.
You can use the code below to retrieve stored cookies. In this case, the stored "auth-cookie".
In this code,
cookie, err := c.Cookie("auth-cookie") retrieves the "auth-cookie" from the request, and returns the cookie value and an error (if any). If there's an error, it returns a "No cookie provided" message and aborts the process.
If there is no error, it means there is a cookie. It returns the cookie and continues the next request chain.
See Also: XSS Attack and How to Prevent it
The complete code.
You should create a Golang local module(controller) which will be used to separate the Login and GetAuthUser functions from the project's main entry point.
Usage:
In main.go file.
In this code, both the LogIn and GetAuthUser local modules are using the GET HTTP METHOD.
Open the main.go file directory in the terminal, and run (go run .).
Test the implementation in any API testing tool (Postman in this case).
- To set the cookies: GET method, and enter the url: localhost:8080/login. This will return a "success" JSON message.
- To get the cookies, GET method, and enter the url: localhost:8080/getAuthUser. This will return the stored cookie as JSON data.
How to Test Your Golang Cookies Implementation.
Testing your cookie implementation:
1. Open your terminal and navigate to your project directory
2. Run `go run main.go` to start the server
3. Use an API testing tool like Postman to test your endpoints.
Setting the cookie:
- Make a GET request to `http://localhost:8080/login`
- You should receive a "success" response.
- Check the Cookies tab in Postman to verify the cookie was set.
See Also: Input Validation and Its Importance in Software Development
Using the cookie:
- Make a GET request to `http://localhost:8080/auth-user`
- The cookie should be automatically sent with your request.
- You should receive the cookie data in the response.
How to Ensure Security Best Practices for Cookies.
Secured cookies are critical for protecting user data and your application. Here are key best practices to follow:
Use the HttpOnly Flag
Always set the HttpOnly flag to `true` to prevent client-side scripts from accessing cookies. This significantly reduces the risk of Cross-Site Scripting (XSS) attacks.
```go
c.SetCookie("auth-cookie", token, maxAge, "/", domain, secure, true)
// ↑
// HttpOnly flag
Set the Secure Flag in Production
Set `secure: true` in production to ensure cookies are only transmitted over HTTPS connections, protecting them from interception during transit.
```go
// Development only
c.SetCookie("auth-cookie", token, maxAge, "/", "localhost", false, true)
// Production
c.SetCookie("auth-cookie", token, maxAge, "/", domain, true, true)
// ↑
// Secure flag
Implement SameSite Protection
Use SameSite attribute to prevent cookies from being sent with cross-site requests, protecting against Cross-Site Request Forgery (CSRF) attacks.
```go
c.SetSameSite(http.SameSiteStrictMode)
```
Set Appropriate Expiration Times
Use short expiration times for sensitive cookies like authentication tokens.
```go
// 5 minutes for authentication
c.SetCookie("auth-cookie", token, 300, "/", domain, secure, httpOnly)
Limit Cookie Data
Store the minimum necessary data in cookies to reduce request size and exposure risk.
```go
// Do this
c.SetCookie("auth-cookie", "jwt_token_here", maxAge, "/", domain, secure, httpOnly)
// Not this
c.SetCookie("auth-cookie", "username=john&email=john@example.com&role=admin", maxAge, "/", domain, secure, httpOnly)
```
Conclusion
In this blog post, you learned about HTTP cookies and how it's used for security (authentication) purposes, storage of users' preferences, and others.
You also learned how to set and get cookies using the Gin package - Golang web framework.
Also, ensuring cookie security is critical for securing user data and preserving user preferences, and the website functionality. Best practices include the following:
- Utilizing the HttpOnly flag to prevent client-side scripts from accessing cookies, hence reducing the danger of cross-site scripting (XSS) attacks.
- The Secure flag ensures that cookies are exclusively transmitted via HTTPS only, protecting them from interception during transit.
- The sameSite attribute prevents cookies from being delivered with cross-site requests, which helps to protect against cross-site request forgery (CSRF) attacks.
- It is important to limit the amount of data kept in cookies and specify proper MaxAge (expiration) to avoid needless data retention. This improves both security and performance by lowering the size of HTTP requests
By understanding these concepts, you are ready to implement authentication cookies in your web applications. Thank you for reading, and don't forget to apply these best practices in your projects.
Please Share